If you reached this blog, know that I no longer write here, but moved to Medium instead:
https://medium.com/@theyonibomber
Please follow me there!
The Code Sheriff
Keeping the streets clean of bad development practices.
Nov 20, 2017
May 29, 2015
Importing Swift code from Objective-C in a Test Target - It's Possible!
TL;DR
By changing the 'PRODUCT_MODULE_NAME' build setting + adding XCTest.h import in the bridging header file, I was able to make this work.
On my previous post about Swift, I mentioned that one of the biggest issues I had with Swift is the fact I get a compilation error on my unit tests target if I ever use the import "MyProject-Swift.h" in a file that is included in this target (whether it's a test case or production code).
I also mentioned this is a big issue in the Swift talk I gave in Reversim Summit 2015 (in Hebrew).
Well, turns out I was wrong (or at least - it can be fixed).
To fully understand the solution, lets' first make sure you understand the problem and why this is such a big issue for me.
I'll try to show a concrete example so you could understand it, and maybe because all names are stupid it may be harder to relate to, but believe me - if you write new swift code in a real life Objective-C project, you will eventually need to use it SOMEWHERE from your Objective-C code.
Here goes:
Lets' say I have an existing Objective-C project with unit tests.
Class A is written in Objective-C and has a unit test.
It also internally uses a class called B, written in Objective-C and doesn't have a unit test.
I now decided I want to add a new class named C. It's written in Swift and I add a unit test for it in Swift.
Now I want to use class C in class B.
I need to add "MyProject-Swift.h" to B's code for it to work, right?
Right.
So here's what we've come up with by now:
If I build and run my app, everything is fine.
If I build and run my unit tests target, I get the following error:
When I originally found out about this (somewhere during the early days of Xcode 6), I was helpless and devastated.
There were only 3 workarounds I could think of and apply to my projects:
This essentially meant that I used swift only for very isolated features in my project, which was very upsetting.
The reason I originally came to the conclusion that it can't be fixed and there aren't any other workarounds, apart from banging my head for a long time about it without success, was an answer on SO basically saying that Apple declared it's a known issue.
In a weird coincidence, just several hours before I decided I should tackle this issue again, a new answer was posted to the same question. It didn't quite solve my problem (I think it's because I didn't move to Xcode 6.3 yet for the problematic project), but it gave me the hope and the direction to a solution!
The Solution
What essentially happens, is that the name of the generated header file that contains all the exported classes from Swift to Objective-C, is called "${PRODUCT_MODULE_NAME}-Swift.h"
What's ${PRODUCT_MODULE_NAME}? By default, Xcode sets it to be the same as the ${TARGET_NAME}, meaning: MyProject-Swift.h for the App target, and MyProjectTests-Swift.h for the test target.
So, we could use some #ifdef statements each time we want to include the generated Swift header, but this is a terrible solution. Instead, I manually changed the product module name the following way:
That's it. For the example project I created for this post it was all I needed to do for solving the problem.
In my real project however, possibly because it was created with an older version of Xcode, I had another issue that I thought was worth mentioning - an issue of Xcode suddenly not running my Swift unit tests (CTest.swift in this example). It was complaining about not finding XCTestCase in the generated MyProject-Swift.h file.
What eventually solved it for me, is adding an XCTest import in my "MyProjectTests-Bridging-Header.h" file in the following way:
And that's it - problem solved.
BTW
If you are having problems with the other way around (Swift code importing Objective-C code in Unit Tests), I recommend reading the following post.
Also, if you have found this post interesting, you should follow me on twitter and listen to my podcast (in Hebrew).
By changing the 'PRODUCT_MODULE_NAME' build setting + adding XCTest.h import in the bridging header file, I was able to make this work.
On my previous post about Swift, I mentioned that one of the biggest issues I had with Swift is the fact I get a compilation error on my unit tests target if I ever use the import "MyProject-Swift.h" in a file that is included in this target (whether it's a test case or production code).
I also mentioned this is a big issue in the Swift talk I gave in Reversim Summit 2015 (in Hebrew).
Well, turns out I was wrong (or at least - it can be fixed).
To fully understand the solution, lets' first make sure you understand the problem and why this is such a big issue for me.
I'll try to show a concrete example so you could understand it, and maybe because all names are stupid it may be harder to relate to, but believe me - if you write new swift code in a real life Objective-C project, you will eventually need to use it SOMEWHERE from your Objective-C code.
Class A is written in Objective-C and has a unit test.
It also internally uses a class called B, written in Objective-C and doesn't have a unit test.
I now decided I want to add a new class named C. It's written in Swift and I add a unit test for it in Swift.
Now I want to use class C in class B.
I need to add "MyProject-Swift.h" to B's code for it to work, right?
Right.
So here's what we've come up with by now:
If I build and run my app, everything is fine.
If I build and run my unit tests target, I get the following error:
![]() |
'MyProject-Swift.h' file not found |
When I originally found out about this (somewhere during the early days of Xcode 6), I was helpless and devastated.
There were only 3 workarounds I could think of and apply to my projects:
- Not importing "MyProject-Swift.h", and therefore having to use tricks like NSClassFromString(@"C") and performSelector - UGLY as hell
- Removing B.m from the Test target (by unchecking the target in the "Target Membership" section of the File Inspector) - Might work for certain cases, but as you see in my example, this means ATest can't compile as it uses A that uses B (that uses Swift code). So if I have enough coverage in my unit tests this is hopeless.
- Porting the whole project to Swift - Too expensive.
This essentially meant that I used swift only for very isolated features in my project, which was very upsetting.
The reason I originally came to the conclusion that it can't be fixed and there aren't any other workarounds, apart from banging my head for a long time about it without success, was an answer on SO basically saying that Apple declared it's a known issue.
In a weird coincidence, just several hours before I decided I should tackle this issue again, a new answer was posted to the same question. It didn't quite solve my problem (I think it's because I didn't move to Xcode 6.3 yet for the problematic project), but it gave me the hope and the direction to a solution!
The Solution
What essentially happens, is that the name of the generated header file that contains all the exported classes from Swift to Objective-C, is called "${PRODUCT_MODULE_NAME}-Swift.h"
What's ${PRODUCT_MODULE_NAME}? By default, Xcode sets it to be the same as the ${TARGET_NAME}, meaning: MyProject-Swift.h for the App target, and MyProjectTests-Swift.h for the test target.
So, we could use some #ifdef statements each time we want to include the generated Swift header, but this is a terrible solution. Instead, I manually changed the product module name the following way:
![]() |
PRODUCT_MODULE_NAME = "MyProject" |
That's it. For the example project I created for this post it was all I needed to do for solving the problem.
In my real project however, possibly because it was created with an older version of Xcode, I had another issue that I thought was worth mentioning - an issue of Xcode suddenly not running my Swift unit tests (CTest.swift in this example). It was complaining about not finding XCTestCase in the generated MyProject-Swift.h file.
What eventually solved it for me, is adding an XCTest import in my "MyProjectTests-Bridging-Header.h" file in the following way:
![]() |
Add #import <XCTest/XCTest.h> to MyProjectTests-Bridging-Header.h
|
And that's it - problem solved.
BTW
If you are having problems with the other way around (Swift code importing Objective-C code in Unit Tests), I recommend reading the following post.
Also, if you have found this post interesting, you should follow me on twitter and listen to my podcast (in Hebrew).
Dec 1, 2014
Why I think Swift is not ready yet
UPDATE Feb '15: With Xcode 6.3, Apple fixed some of the issues this post is talking about. However - there are still some major issues for me, the number one of them is the fact I can't use Swift classes in Objective-C without porting the tests for them to Swift.
Like every relatively-early-adpoter-iOS-developer, I decided to give Swift a try in the past few features I worked on for Piano Maestro.
It was a lot of fun to get into, and I wrote some pretty code and features came out very nice. However, I ran into some issues, that made me eventually come to the conclusion that swift isn't quite ready yet, and I should stick to Objective-C as the main language for developing iOS apps. At least for now.
Here are my top reasons:
Inaccuracies in compilation errors
Often, when a line is relatively complex and has a compilation error, compiler will give you an error message that's simply not describing the real issue.
The most common use case is a simple error like sending the wrong primitive type as an argument to a function (a problem that happens a lot to us Objective-C/C/C++ developers, which are used to implicit conversion of primitives).
Consider the following example:
WAT??
dispatch_after receives exactly these types arguments. So what's wrong here?
Turns out the problem is that dispatch_time expects Int64 to be sent as the second argument, and I actually sent NSTimeInterval.
But how could I know that from that irrelevant error message?
There went an hour of banging my head until I figured this out.
UPDATE Feb '15: at least for this example, was fixed in Xcode 6.3 Beta 1 and error message is now very descriptive and relevant.
Debugging is hard
More head banging often happened when trying to see the value of a variable in the debugger, I eventually find myself adding debug prints instead.
A tip about this: If you get "Some" as the output of trying to 'po var', you can 'po print(var)' instead, and it will (hopefully) work.
Can't use Swift code within Objective-C in unit tests
Update May 2015: I found workaround!
While it's absolutely possible to mix Swift <-> Objective-C code in the app's main target (apart for some limitations I will rant about later), in the test target, I can't import $(PRODUCT_MODULE_NAME)-Swift.h in Objective-C code.
While it's absolutely possible to mix Swift <-> Objective-C code in the app's main target (apart for some limitations I will rant about later), in the test target, I can't import $(PRODUCT_MODULE_NAME)-Swift.h in Objective-C code.
This does not only mean that tests for Swift classes can only be written in Swift (fair enough), it also means that any Objective-C code in my app that uses Swift classes cannot be tested at all. Build will fail if it's included in the test target of the project.
LAME.
See:
Can't use tuples and trivial enums in Objective-C
As the official documentation says, you can't use Swift-only features in your Objective-C code.
This makes sense for some things, but it would make a huge difference if they could have made an effort to expose some of the more trivial features in the $(PRODUCT_MODULE_NAME)-Swift.h bridge:
- Trivial enums can become C enums with a prefix (like it's done in the opposite direction)
- Tuples can become trivial classes
etc.
For now, I can't expose functions that receive a tuple or a Swift enum to Objective-C, and must add some kind of bridge myself (e.g. get an Int parameter instead of an enum and use the constructor with rawValue:).
For now, I can't expose functions that receive a tuple or a Swift enum to Objective-C, and must add some kind of bridge myself (e.g. get an Int parameter instead of an enum and use the constructor with rawValue:).
Maybe it was too much of an effort for Apple to deal with, but this makes it harder to use these features if I know the class will be used in Objective-C code.
UPDATE Feb '15: you can now use trivial enums!
Can't use c++ in Swift
If like us, your app uses Objective-C++ code and not only Objective-C, you're in trouble and won't be able to use any code that uses c++ types in the header. You'll have to create some kind of Objective-C bridge that will hide the c++ types.
See: http://stackoverflow.com/questions/24042774/can-i-mix-swift-with-c-like-the-objective-c-mm-files
Can't use mocking frameworks
Because of limitations in the availability of the language runtime in Swift, there's no good way to use a mocking framework for tests right now. That's a big problem.
In the meanwhile - best workaround IMO is adding an Inner Class called "MockMyClass" in your test, and overriding any methods you want to mock (like we did in 2006 before mocking frameworks were introduced).
See:
Xcode sucks, AppCode has only limited support
So, as you probably know from my Xcode vs AppCode comparison (a bit outdated, but most of it still applies), I don't use Xcode for coding. It's lacking some very basic IDE mechanisms that AppCode is excellent at (e.g. Extract Method, Quick Fix, Find Usages, ...).
This is still true for Xcode 6.1 and Swift support. Sure, Xcode improved since writing this comparison, but still - I can't even extract method? This sucks.
Unfortunately, Swift support in AppCode is still very rudimentary. But the good news is that they are working on it.
In the meanwhile, here's a link to AppCode EAP that contains some basic features of syntax highlighting and some basic refactoring in Swift. I still don't recommend it over Xcode's current Swift support though.
UPDATE Feb '15: AppCode 3.x has basic Swift support, but still has a very long way to go in order to be as good as it is for Objective-C in terms of refactoring etc.
UPDATE Feb '15: AppCode 3.x has basic Swift support, but still has a very long way to go in order to be as good as it is for Objective-C in terms of refactoring etc.
To sum it up
I guess things are not that bad, because Swift is a very neat language and has some really cool features I'm in love with, like tuples, better enums, cooler closures, generics, property observers, and many many more.
I assume my complaints are either going to be solved soon, or become less of an issue - many of them are mix&match issues, which only affect apps with existing Objective-C/C++ code. Hopefully
Anyhow, at least for the time being, I guess I'll stick with Objective-C as the main iOS development language.
Further reading:
Developing a Bidding Kiosk for iOS in Swift - a great retrospective with some more reasons of why Swift isn't ready yet.
Mar 13, 2014
8 Tips for working effectively with Interface Builder
Introduction
While working at JoyTunes on the latest versions of our top-notch piano app, we've done a great deal of UI redesign and therefore had to spend long hours with the notorious Interface Builder, resizing images and views, which can be a lot of frustrating work.
While working at JoyTunes on the latest versions of our top-notch piano app, we've done a great deal of UI redesign and therefore had to spend long hours with the notorious Interface Builder, resizing images and views, which can be a lot of frustrating work.
During this work, we actually discovered a great deal of neat IB tricks, and I decided I must share them with the world.
So I conducted this little post of my 8 greatest tips for working effectively with IB.
A little disclaimer:
In JoyTunes, we work with .xib files (not storyboards), and without Auto Layout. This is mostly for historical reasons. As a result, some of the tips I'm mentioning might react a little differently for you if you're using storyboards or Auto Layout, but most should apply just the same.
1. Size to Fit Content
I'm so ashamed I only took the effort to look this up only recently. A HUGE time saver.
Select any view and then go to Editor->Size to Fit Content or simply press ⌘=
Will resize the selected view to fit its content in the following manner:
- An ImageView/Button will be resized to the image's original size (most common usage):
- A Label/Button will be resized to fit the text it's currently containing:
- A parent container view to fit the frames of its subviews.
2. Holding option key to view distance from another view's edges
Holding option key after selecting a view, and then hovering over some other view, will show you cool distance indicators from that view's edges:
3. Editor -> Embed In View, Unembed:
Do you know that annoying feeling when you have some subviews all located and then you decide they need to have a different parent view, or even a different .xib, and then they are all automatically get located in the center of the new view?
Well - here's the solution:
4. Adding padding to freeform sized view without affecting subview's positions
When trying to add padding to a view, the x's and y's of the subview is retained, which isn't always what you want.
The best way I found to get past this, is to drag the edges while holding ⌘:
When trying to add padding to a view, the x's and y's of the subview is retained, which isn't always what you want.
The best way I found to get past this, is to drag the edges while holding ⌘:
5. Move a view that isn't in the front:
One thing that bugs me when working with IB, is the inability to move views that aren't in front easily.
One way to do so will be to temporary bring it to front and then bring it back, but this is annoying.
Another way will be to use the size inspector on the right panel, which is fine but could take some guessing and adjustments until you get it right.
A way I found of moving a view with the arrow keys without bringing it to front is this:
1. Choose the view on the document outline.
2. Click on the frame of the root view in order to gain focus.
3. Move with arrow keys.
EDIT:
Some other (better) way of gaining focus, taken from the comments to this post:
One thing that bugs me when working with IB, is the inability to move views that aren't in front easily.
One way to do so will be to temporary bring it to front and then bring it back, but this is annoying.
Another way will be to use the size inspector on the right panel, which is fine but could take some guessing and adjustments until you get it right.
A way I found of moving a view with the arrow keys without bringing it to front is this:
1. Choose the view on the document outline.
2. Click on the frame of the root view in order to gain focus.
3. Move with arrow keys.
EDIT:
Some other (better) way of gaining focus, taken from the comments to this post:
- If you double-click a view in the outline, it will switch focus to the layout pane with that view selected.
- Shift+Right(Ctrl) Click over the view in IB and you'll see a menu of all the views that exist where you've clicked.
6. IBOutletCollection ordering
Often, the order of IBOutletColletions is important to us, and we want to iterate on them in code in that order.
One way of doing it is sorting them in code by their x/y/tag, and then iterate.
This is unnecessary. The order of connection is determined by the order in which we dragged the connection, and we can see the current order if by ⌃+Clicking the File's Owner:
7. Use custom properties
I think a relatively underused feature of IB is the ability to set custom properties on views, using the "User Defined Runtime Attributes" of the Identity inspector:
As you can probably guess, we defined a JTLabel class that you can set stroke color and width to, and this is our way of immediately setting this without having to write any code.
Another common hack that can be applied this way is setting "layer.cornerRadius" of any view, to make it have rounded corners.
A relevant tool that uses this mechanism is Canvas, which will let you define animations for views without writing code.
Important warning regarding this that saved my life on one occasion:
One way of doing it is sorting them in code by their x/y/tag, and then iterate.
This is unnecessary. The order of connection is determined by the order in which we dragged the connection, and we can see the current order if by ⌃+Clicking the File's Owner:
7. Use custom properties
I think a relatively underused feature of IB is the ability to set custom properties on views, using the "User Defined Runtime Attributes" of the Identity inspector:
As you can probably guess, we defined a JTLabel class that you can set stroke color and width to, and this is our way of immediately setting this without having to write any code.
Another common hack that can be applied this way is setting "layer.cornerRadius" of any view, to make it have rounded corners.
A relevant tool that uses this mechanism is Canvas, which will let you define animations for views without writing code.
Important warning regarding this that saved my life on one occasion:
In your implementation class you cannot use initWithCoder: otherwise your key-value path setting will not be picked up. You will have to do all your implementation within awakeFromNib.
(Credit to http://www.wannabegeek.com/?p=395)
8. MoarFonts - custom fonts WYSIWYG
Custom fonts have always been a big issue when working with Interface Builder.
There's no built-in support for those, and the best solutions I have seen were either using custom properties solutions like Canvas, or using font replacing techniques like IBCustomFonts. These are valid solutions, but they have a great downside - they don't give you a full WYSIWYG experience, which is exactly why you are using Interface Builder in the first place.
It can be very annoying to place a label with a placeholder font and then having to run the app in order to discover that the width you gave it doesn't fit well with your replacing custom font.
Lately I stumbled upon this solution called MoarFonts. It costs 10$, and there's no demo or trial - but trust me, it's totally worth it!
It's a pretty simple setup: you'll have to build your app with MoarFonts as a script build phase, restart Xcode, and voila! You can see custom fonts in InterfaceBuilder:
That's it!
I hope at least some of the tips were something you didn't know.
If you have any other tips you think I should add to the list, or if you have a better way to achieve some of the stuff I was trying to solve, please send me a tweet (@theyonibomber).
Also, you should listen to my podcast about mobile development & beer (in Hebrew).
Sep 26, 2012
Using Crittercism SDK to effectively find & fix crashes
This post is talking about iOS apps, but I'm sure most of it applies to Android as well...
Having a crash report framework for your app is not really optional.
While investing in the QA phase before submitting your app can find and eliminate a lot of the crashes, there will always be something you'll miss, and there's nothing like millions of potential users to help you find and fix these annoying crashes when they happen.
The framework is really simple to use and the documentation is very succinct, so I'm not going to elaborate too much about the basic integration and features (such as symbolicated stack traces for each crash), but I DO want to tell you about some of my favorite features in the framework, that got us at JoyTunes to choose and use it for our iOS apps.
Apr 14, 2012
Developing "Piano Dust Buster"
So... "Piano Dust Buster" - the game I've been working on in the past few months in JoyTunes - is live in the iPad App Store for two weeks now. This is very exciting to me, so I decided to write a blog post about it, sharing some thoughts about this game and how it was to develop it.
The idea is to give a tap-tap/guitar hero like experience, while being on the more educational side (e.g. piano keys are real-life sized + there's a game mode with a musical staff that you can learn reading sheet music through) + instead of playing with a toy guitar, you can play with your own real instrument, so you're actually practicing.
Here's the game trailer, so you can get a hunch of what's the game like:
For more info, visit the game's homepage: www.joytunes.com/piano
The link to the game: http://itunes.apple.com/us/app/piano-dust-buster-song-game/id502356539?mt=8
About the game
The game is a piano song game, that you can play both in touch mode and with your own real piano.The idea is to give a tap-tap/guitar hero like experience, while being on the more educational side (e.g. piano keys are real-life sized + there's a game mode with a musical staff that you can learn reading sheet music through) + instead of playing with a toy guitar, you can play with your own real instrument, so you're actually practicing.
Here's the game trailer, so you can get a hunch of what's the game like:
For more info, visit the game's homepage: www.joytunes.com/piano
The link to the game: http://itunes.apple.com/us/app/piano-dust-buster-song-game/id502356539?mt=8
Feb 4, 2012
Xcode vs. AppCode
Some Background
(mostly some personal info about me - skip if you're only interested in the comparison):
I've always been an Eclipse fan.
Eclipse was always my favorite IDE for Java, and therefore when I started developing in Python PyDev was the obvious and excellent choice.
I tried a little bit of Jetbrains' IntelliJ and PyCharm. They both seemed very impressive, but to be honest - whole the project settings there were a little bit scary for me, plus they cost money and I was happy with the free Eclipse so I didn't see a good reason to make the move.
Upon joining JoyTunes, I started to develop in 2 new technologies for me: ActionScript3, and Objective-C.
Dec 28, 2011
Excuse #6 - We are such good programmers, we don't need tests!
Welcome to the 6th excuse in the Testing: Why Bother? series.
I'm going to address this excuse a little bit differently than the other ones and be more personal about it.
Reading the headline of this excuse may sound dubious to some of you, but I assure you it's not something I made up. I actually heard this excuse while I was looking for a job a while back and was interviewing for a startup company.
I'm going to address this excuse a little bit differently than the other ones and be more personal about it.
Reading the headline of this excuse may sound dubious to some of you, but I assure you it's not something I made up. I actually heard this excuse while I was looking for a job a while back and was interviewing for a startup company.
Dec 12, 2011
Excuse #5 - The Frequent Refactoring Excuse
Welcome to the 5th excuse in the Testing: Why Bother? series:
"We refactor our code so frequently, that the time we invest in tests just isn't worth it - they are going to change and be irrelevant anyhow"
This excuse is one of my favorites, because it's so ironic, yet surprisingly quite common.
"We refactor our code so frequently, that the time we invest in tests just isn't worth it - they are going to change and be irrelevant anyhow"
This excuse is one of my favorites, because it's so ironic, yet surprisingly quite common.
Nov 4, 2011
Excuse #4 - Running the tests takes forever
So, after a long break (been focusing my time on reading about new technologies rather than writing - started a new job @ JoyTunes...) I'm back with the next excuse in the "Testing, Why Bother?" series.
"Running the tests takes too much time,
so we never run them anyhow."
Well, this excuse is less common than the other ones on the list, and to tell the truth - if that's your case, you're in a pretty good spot - because, at least you have tests to run!
However, if you have tests and you're not running them, they obviously have little merit, so the effort of writing them goes to waste, which is definitely a shame.
So here are a few tips on how you can solve this tricky situation.
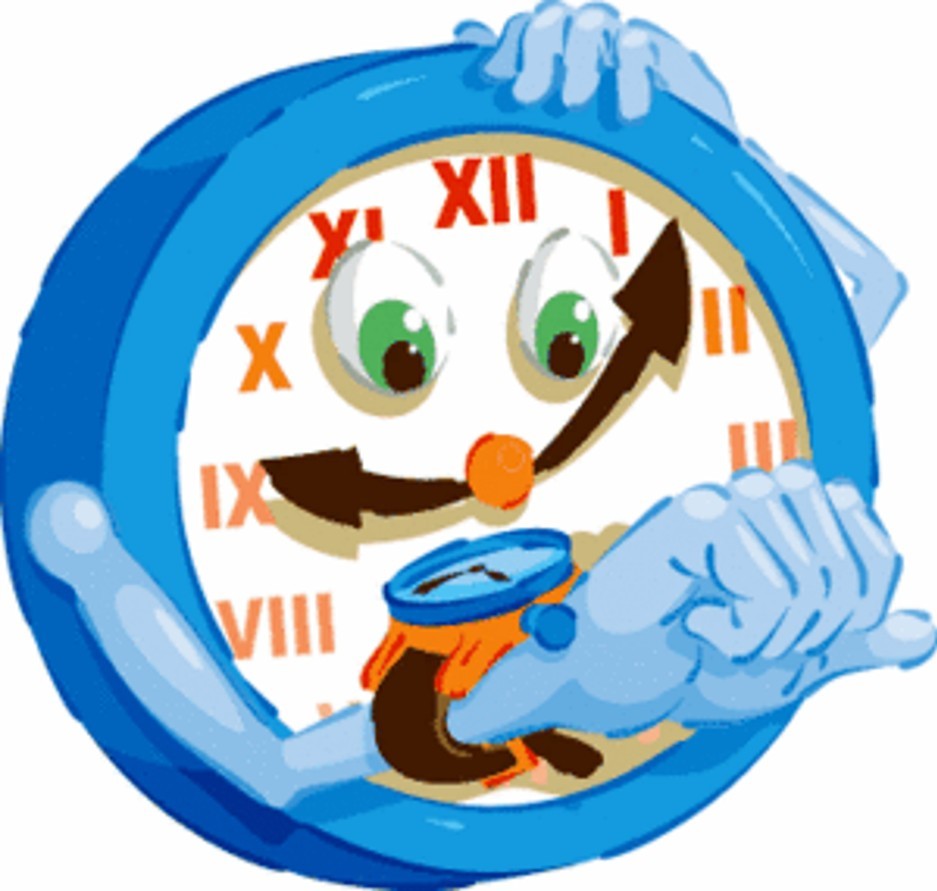
so we never run them anyhow."
Well, this excuse is less common than the other ones on the list, and to tell the truth - if that's your case, you're in a pretty good spot - because, at least you have tests to run!
However, if you have tests and you're not running them, they obviously have little merit, so the effort of writing them goes to waste, which is definitely a shame.
So here are a few tips on how you can solve this tricky situation.
Aug 22, 2011
TDD and Unit-Testing for Python Developers
A few days ago, I gave a 1:30 hours long talk (in Hebrew) in my company about TDD and Unit-Testing for python developers.
The idea was to focus both on what is TDD, including a 45 minutes demo, and to give some concrete tips for unit-testing in python.
Usually when I give a talk about TDD, I use some kind of simple kata as a demo, like the prime factors kata of Uncle Bob. The problem with that, is I usually get responses like "TDD works only for calculators examples". I tried to be a little more original here and demonstrate a more real-life like example, and I tried not to come prepared too much, so I could demonstrate a genuine way of thinking about the design and letting it drive my code.
Looking back, I think this caused the demo to be a little messy - I told the audience to participate and throw ideas in the middle, and this caused things to advanced a little bit too slowly, and we didn't really get to real value by the end of the demo (as opposed to using a simple kata when you get value by the end of 5-10 tests).
For the tips sections, I took most of the material from Michael Foord's great blog and mocking framework: http://www.voidspace.org.uk/, and I think it should be very useful. So thank you for that Michael!
Anyhow, apart from the messy demo - which I think is still educational - I feel like the talk went fine, and decided to share it.
The Talk
Here's an unedited screen and sound capture of the talk, for the Hebrew speakers of you.
If you can't watch it through this shaky flash plugin, you can download it from here
The idea was to focus both on what is TDD, including a 45 minutes demo, and to give some concrete tips for unit-testing in python.
Usually when I give a talk about TDD, I use some kind of simple kata as a demo, like the prime factors kata of Uncle Bob. The problem with that, is I usually get responses like "TDD works only for calculators examples". I tried to be a little more original here and demonstrate a more real-life like example, and I tried not to come prepared too much, so I could demonstrate a genuine way of thinking about the design and letting it drive my code.
Looking back, I think this caused the demo to be a little messy - I told the audience to participate and throw ideas in the middle, and this caused things to advanced a little bit too slowly, and we didn't really get to real value by the end of the demo (as opposed to using a simple kata when you get value by the end of 5-10 tests).
For the tips sections, I took most of the material from Michael Foord's great blog and mocking framework: http://www.voidspace.org.uk/, and I think it should be very useful. So thank you for that Michael!
Anyhow, apart from the messy demo - which I think is still educational - I feel like the talk went fine, and decided to share it.
The Talk
Here's an unedited screen and sound capture of the talk, for the Hebrew speakers of you.
If you can't watch it through this shaky flash plugin, you can download it from here
Aug 2, 2011
Getting started with iOS development
So... In the last couple of weeks, I've been researching iOS development - reading some blogs, going to some lectures or watching them on iTunes U, searching for answers to common questions on stackoverflow, etc.
This doesn't make me an expert on the subject, but I think if like me - you're thinking about starting with iOS development or mobile development in general, this post might save you some time and make sure you're not missing any of the important technologies available today (August 2011), and the information you need to know in order to get started.
Before You Start...
Do I have to own a Mac in order to develop for iOS?
This doesn't make me an expert on the subject, but I think if like me - you're thinking about starting with iOS development or mobile development in general, this post might save you some time and make sure you're not missing any of the important technologies available today (August 2011), and the information you need to know in order to get started.
Before You Start...
Do I have to own a Mac in order to develop for iOS?
- In a word: YES.
- There might be frameworks that will let you develop the applications without a Mac, but when you'll want to deploy on a real device, or to the app-store, you're going to need a Mac for that.
- Even if you succeeded in creating a Hackintosh on a VM (I personally had a really hard time trying to do so...), it is not recommended - as you are going to have troubles once you'll need to update your software/OS version, etc.
- I tried to find online solutions for "Mac for rent", and found macincloud.com, but 2 days after sending a request for a trial they announced they closed the site for new users.
Jul 27, 2011
Excuse #3 - Isn't it QA's job to test my code?
Sorry for the delay in publishing this one guys, had been busy, but finally - the 3rd excuse of the "Testing: Why Bother?" series is finally up.
So... We are going to address an excuse that is a bit less commonly asked out loud, but I think many of us programmers secretly think it without even knowing.
I assume you stumbled at least once before across arguments like:
- "Well... I could write a test for this, but QA has to perform some heavy testing on this feature anyhow, so why bother?"
- "I don't have the environment I need to run a test for this right now, and QA have their own testing environment, it would be much more cost-effective to just leave the job of testing this to them"
- "Our QA guys are not doing anything right now anyhow... We are on a lot of pressure and have tons of new features to implement before deadline - why not speed up things a bit by skipping the testing part and leaving it to QA?"
Well, I must admit these are all thoughts that ran through my head at least once or twice.
Here are some of my key points on what I've come to learn from my experience with other programmers and even myself cutting corners about testing the code because we're on a hurry and "QA are going to test it anyhow":
Jun 22, 2011
A test with no assert is better than no test at all
Sorry about the delay since my last post.
Now that I've freed some spare time, I hope to get back to the "Testing: Why Bother?" series. So stay tuned.
Anyhow, wanted to share a quick insight I had about what to do when you need quick feedback about a feature that you find hard to write a good test for, through an example.
Some Background
I was pairing with my buddy on a cool feature of our project for some time now.
The feature is basically some kind of a "Device Prettifier", that receives a local device path, performs some inquiries about it, and when its __repr__ (toString() equivalent) is called, it displays all the data it gathered from the inquiries prettily.
So, we actually test-driven-developed the mentioned DevicePrettifier, unit-testing everything by creating mock devices returning whatever we want the DevicePrettifier to do. Basic TDD+Mocking, went very smoothly and was a lot of fun.
Now that I've freed some spare time, I hope to get back to the "Testing: Why Bother?" series. So stay tuned.
Anyhow, wanted to share a quick insight I had about what to do when you need quick feedback about a feature that you find hard to write a good test for, through an example.
Some Background
I was pairing with my buddy on a cool feature of our project for some time now.
The feature is basically some kind of a "Device Prettifier", that receives a local device path, performs some inquiries about it, and when its __repr__ (toString() equivalent) is called, it displays all the data it gathered from the inquiries prettily.
So, we actually test-driven-developed the mentioned DevicePrettifier, unit-testing everything by creating mock devices returning whatever we want the DevicePrettifier to do. Basic TDD+Mocking, went very smoothly and was a lot of fun.
Apr 5, 2011
Excuse #2 - Writing tests is too hard
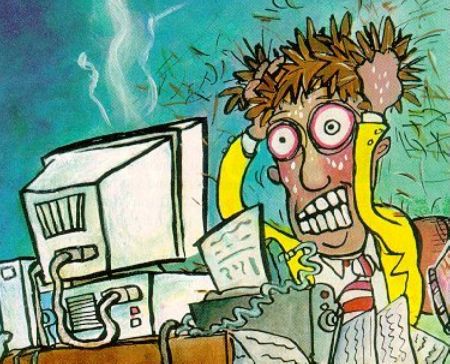
So, after talking about the famous too-much-time excuse, I am going to address the next excuse in the Testing: Why Bother? series: We don't write tests because it's too hard!
I will try to make this post a little more practical than the previous one, but because it's still aimed more to the why and not the how, I'm not going to dive too much into the "how to write tests for beginners" techniques. If you feel you need some prior background for this, I can recommend the following great books:
![]() | ![]() | ![]() |
So... I guess in your pretty world testing is a piece of cake...

Mar 29, 2011
Excuse #1 - Writing tests takes too much time
This is the most common excuse in the "Testing: Why Bother?" series, so I'm going to address it first.
It turned out to be a pretty long post (and only the second of several more), but I really think if you'll read everything through it will give you some new insights.
So... are you claiming testing doesn't take time?
Well... Of course I'm not. Testing is not free, and you don't HAVE to write automated tests in order to write code that eventually works. But is it really not cost-effective?
So lets' dig in and elaborate on all of these points.
It turned out to be a pretty long post (and only the second of several more), but I really think if you'll read everything through it will give you some new insights.
So... are you claiming testing doesn't take time?
Well... Of course I'm not. Testing is not free, and you don't HAVE to write automated tests in order to write code that eventually works. But is it really not cost-effective?
When I demonstrate unit-testing or TDD to developers new to the field, I frequently get reactions such as "well, that's nice, but I could have implemented the same code much faster without tests."
This statement might be true, but there are several reasons why I think by testing you actually save time:
- You don't have to start thinking how to verify that your code works (you already did this)
- You are already after the debugging phase
- You ensure having automated tests that can run later on
There's also the concept of improving design and usability of your code by testing it, which may also save you time in future, but that's something I'm going to talk about more in the next posts of this series.
So lets' dig in and elaborate on all of these points.
Mar 27, 2011
Testing: Why Bother? - Introduction
I stumbled upon an interesting discussion this week in the testing-in-python mailing list, asking how to explain why testing is important to a "we do not write tests" audience. Because this is something I had to do a few times already, I thought about writing a blog post about it (actually, a series of posts...)
Testing? What do you mean by that?
Well, there's a lot of things that can be related as "testing" when talking about software development. There's TDD, Unit tests, Integration tests, having a QA engineer test your code, and more.
In these posts, I'm talking about justifying writing any kind of automated tests that can be run through a CI (Continuous Integration) system after each commit. It doesn't matter if they were originally written during practicing TDD, the important thing is making sure there's something that's always there to test nothing was broken after each little changeset in the code.
So, what's the deal? Why do I need to write tests?
Well, the advantages of testing your software are usually clear and aren't really debatable.
Every one will agree that when you write and run automated tests for your code, you become more confident that your code works, and when you run your tests after each change you make you can gain confidence that you didn't break anything.
The problem is, though these advantages are pretty much obvious and clear to anyone, the majority of developers do NOT write automated tests.
But, if testing is so awesome, why wouldn't everyone write tests?
Well, there are 2 kinds of anti-testing developers (that's how I'm going to refer to them from now on).
The list of excuses
In my experience as a pro-testing preaching developer, I've come across some various types of resistance. I've collected a list of common excuses for justifying the anti-testing approach:
Testing? What do you mean by that?
Well, there's a lot of things that can be related as "testing" when talking about software development. There's TDD, Unit tests, Integration tests, having a QA engineer test your code, and more.
In these posts, I'm talking about justifying writing any kind of automated tests that can be run through a CI (Continuous Integration) system after each commit. It doesn't matter if they were originally written during practicing TDD, the important thing is making sure there's something that's always there to test nothing was broken after each little changeset in the code.
So, what's the deal? Why do I need to write tests?
Well, the advantages of testing your software are usually clear and aren't really debatable.
Every one will agree that when you write and run automated tests for your code, you become more confident that your code works, and when you run your tests after each change you make you can gain confidence that you didn't break anything.
The problem is, though these advantages are pretty much obvious and clear to anyone, the majority of developers do NOT write automated tests.
But, if testing is so awesome, why wouldn't everyone write tests?
Well, there are 2 kinds of anti-testing developers (that's how I'm going to refer to them from now on).
One type of anti-testers, are the kind that simply lack the education. Most programmers don't learn how to write tests as part as their formal education, and therefore when they start at their first software industry job, they usually don't write tests unless exposed to this subject as part of the company's training and mentoring. Because concepts like TDD and CI are relatively new, a lot of old-fashioned team leaders and R&D managers are not exposed to the importance of testing as well, and they don't demand this from new employees and don't include this in training them to the job.
These kind of anti-testing developers are the relatively easy kind. They did not already develop antagonism towards testing, and they may be easily persuaded by fellow pro-testing developers, teammates, managers or external preachers.
The other type of anti-testing developers, is the type that I'm targeting these blog posts towards. These are developers that are already exposed to the concepts, maybe even experimented with testing a little bit in the past, but claim to have some solid reasons why testing is a waste of time for them, and simply do not believe in it. There's usually a list of excuses they give to justify their hostility towards testing.The list of excuses
In my experience as a pro-testing preaching developer, I've come across some various types of resistance. I've collected a list of common excuses for justifying the anti-testing approach:
- Writing tests takes too much time
- Writing tests is too hard
- It's QA's job, and they are going to test our code anyhow
- Running the tests takes forever, so we never run them anyhow
- We frequently refactor everything heavily, all the test code is going to break and be irrelevant anyhow
- We hire only extremely talented developers. We never have bugs
- Even if we'll write tests for everything, we're still going to have bugs
- It's hard to tell how the code behaves each time, so I can't write a test for it
- Writing tests is boooring!
In my next posts, I'll try to attend all of these excuses, and clarify why I think they are plain misconceptions.
Can you think of any more excuses? Please let me know in the comments.
Mar 26, 2011
First Post
Hello,
I'm Yoni Tsafir and I'm a software developer from Israel, currently working at IBM (XIV Storage Systems).
Following the lead of many great minds I appreciate, I decided to start my own blog and share my thoughts.
This blog is going to talk about all these subjects I mentioned, and more. It's going to contain tips I gathered from my experience and try to make it easier for fellow "bad cops of code" like me to explain to their fellow teammates why these concepts are important.
Something else that I have a lot of passion to is music. I'm a music composer, recently composed some TV shows, DVD and musicals for children in Israel. This is something I'll try to take advantage of in order to spice up my posts and share some off-topic interesting content related to the intersection between music and software.
Hope you'll enjoy reading.
Finally, I would like to acknowledge some of the other software related blogs, as they were the main inspiration source I had for starting my own.
I'm Yoni Tsafir and I'm a software developer from Israel, currently working at IBM (XIV Storage Systems).
Throughout my years as a software developer, I commonly found myself in the position where I'm aspiring to find ways of making myself and my teammates work better and more effectively, applying agile practices such as unit-testing, pair-programming, TDD, continuous integration, and so on.
Because of my passion to the subject, insisting of everyone writing an informative commit message, not changing code without making sure there's a test for it first, and so on, in one of my teams I even earned the nickname "The Bad Cop of Code", hence the idea for a name for my blog.
Following the lead of many great minds I appreciate, I decided to start my own blog and share my thoughts.
This blog is going to talk about all these subjects I mentioned, and more. It's going to contain tips I gathered from my experience and try to make it easier for fellow "bad cops of code" like me to explain to their fellow teammates why these concepts are important.
Something else that I have a lot of passion to is music. I'm a music composer, recently composed some TV shows, DVD and musicals for children in Israel. This is something I'll try to take advantage of in order to spice up my posts and share some off-topic interesting content related to the intersection between music and software.
Hope you'll enjoy reading.
Finally, I would like to acknowledge some of the other software related blogs, as they were the main inspiration source I had for starting my own.
- Aviv Ben-Yosef's The Code Dump. Aviv is one of the most, if not the-most talented developers I've ever met, and I even had the pleasure of working and pairing with him for a few years. His blog has some amazing posts and ideas.
- Iftach Bar's Nash Code Bar. Contains an amazing post about hudson/jenkins. Hope to see some more posts like this in the near future!
- Uri Lavi's Blog. Founder of the Software Craftsmanship group in Israel (SCIL).
- Uncle Bob's Blog. Author of Clean Code. Contains excellent videos and posts.
- GeePawHill's Situated Geekery. Some of the bests posts I've read about TDD.
- Lior Friedman's IMistaken. Lior gave a very impressive talk in SCIL, and since then I started reading his very good blog.
- Ran Tavory's PrettyPrint. Ran is a fellow member of SCIL, besides a great podcast (reversim) he runs, he also has a great blog.
Subscribe to:
Posts (Atom)